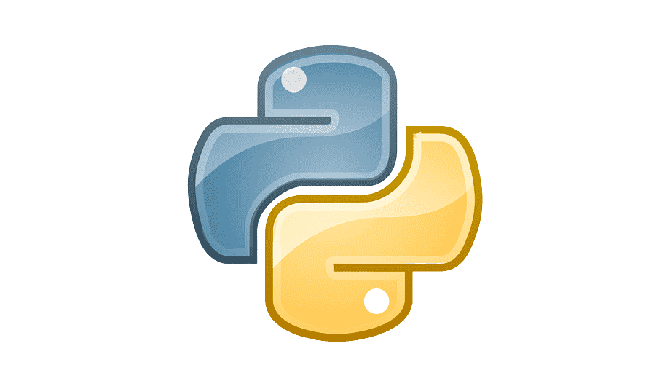
Python iter() function
The iter() function is a built-in function used in Python to convert an Iterable object to an iterator. An Iterable object is an object that contains multiple values. Examples of this trouble object include lists, tuples, strings, and sets. Represents an object that can be retrieved by iterating the elements of an Iterable object one by one.
Basic usage of iter() function
iterator = iter(iterable)
In the code above, Iterable represents the Iterable object that you want to convert to Iterator, and Iterator is an Iterator object that can be used to iterate over Iterable. Using an Iterator, you can use the next() function to get the next element of an Iterable object.
For example, let’s look at an example of converting a list to an Iterator and traversing it.
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list)
print(next(my_iterator)) # 1
print(next(my_iterator)) # 2
print(next(my_iterator)) # 3
Make my_list an iterable object using the iter() function and retrieve the values one by one using the next() function. Now that we have learned how to use the basic iter() function, let’s look at various examples of using the iter() function and see how it is used in various situations.
Convert string to Iterator and traverse it
my_string = "Hello"
my_iterator = iter(my_string)
for char in my_iterator:
print(char)
Those of you who are good at Python or have done Python at least may have noticed, but there is no need to use the iter() function to iterate over a string in a for loop in Python. Basically, iterable objects (e.g. lists, tuples, strings) are iterable because the magic methods __iter__() and __next__() are defined. So, when we iterate over objects such as lists, tuples, and strings with a loop, as in the example above, __iter__() and __next__() are called internally.
Convert tuple to Iterator and traverse it
my_tuple = (10, 20, 30)
my_iterator = iter(my_tuple)
while True:
try:
value = next(my_iterator)
print(value)
except StopIteration:
break
Likewise, tuples are also basically Iterable objects, so they can be iterated in loops without using the iter() function. As mentioned earlier, lists, tuples, strings, etc. are basically Iterable objects, so there is no need to specify the iter() function to iterate. So, the question that arose here was, “Then when is it necessary to use the iter() function?”
Custom iterable object
class MyIterable:
def __init__(self):
self.data = [1, 2, 3]
self.index = 0
def __iter__(self):
return self
def __next__(self):
if self.index < len(self.data):
result = self.data[self.index]
self.index += 1
return result
else:
raise StopIteration
my_iterable = MyIterable()
my_iterator = iter(my_iterable)
for item in my_iterator:
print(item)
When users create an Iterable object by defining their own class, they must use the iter() function. This class defines the __iter__() method, which returns self. This allows the object to operate as an Iterable object.
- The __init__() method is a method that initializes an object. In this method, the data property is created to define the list of data that the Iterable object repeats, and the index property is initialized to 0.
- The __iter__() method must return an Iterable object. Iterable returns self so that the object itself can be iterated.
- The __next__() method is responsible for returning the next element when the Iterable object is repeated. Use index to track the index of the current element, retrieve the element from its data property, and then increment the index. If the index exceeds the data length, the iteration ends by raising a StopIteration exception.
In conclusion, the iter() function can be used to convert any Iterable object (built-in data types as well as user-defined classes or objects) to an Iterator. This function helps in creating an Iterator, and using an Iterator, we can iterate over the elements of an object one by one. Therefore, the iter() function can be useful in various situations.