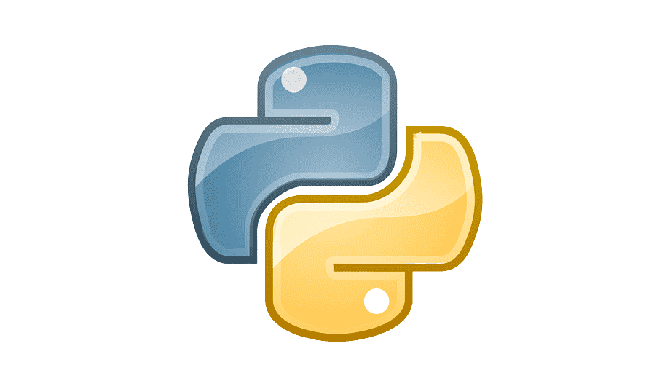
Python try except exception handling
Not only in Python but also in programming, we have all probably experienced situations where the program stops when an error occurs. However, if you do not want the program to stop even if an error occurs, you can use the try except statement in Python. Today, let’s learn how to use the try except statement with various examples.
How to handle exceptions in Python
First, let’s write the code that will cause the error.
print("==== Program begins ====")
x = int('Choi') # Where the error occurs
print(f'my name is {x}')
print("==== Program Ends ====")
Because the string ‘Choi’ cannot be converted to an integer type, an error will occur at the x = int(‘Jack’) line.
==== Program begins ====
Traceback (most recent call last):
File "i:\github\Python\note\note.py", line 3, in <module>
x = int('Choi') # Where the error occurs
^^^^^^^^^^^
ValueError: invalid literal for int() with base 10: 'Jack'
PS I:\github\Python>
As you can see from the execution results above, in Python, if you do not use a separate try except statement, you can see that the program terminates at the point where an error occurred.
Prevent the program from terminating even if an error occurs using the try except statement
In fact, if you write code so that the program dies when an error occurs in a program that the user directly uses, it could be a big problem. Therefore, developers must write code so that the program flows in a different direction even if an error occurs in the most likely place. And this is called exception handling, and it is used to prevent the program from dying due to an error.
Let’s take the code above and handle exceptions using try and except statements.
print("==== Program begins ====")
try:
x = int('Choi') # Where the error occurs
print(f'my name is {x}')
except:
print("==== Error, but program is still alive")
print("==== Program Ends ====")
If you look at the code above, you will get a feel for how to use the try and except statements. In the try statement, you can write code where an error occurs or is expected to occur, and in the except statement, you can write code to handle when an error occurs. Therefore, if you run the above code, you will see the results below.
==== Program begins ====
==== Error, but program is still alive
==== Program Ends ====
What you need to pay attention to here is that as the code x = int(‘Choi’) is executed, an error occurs at that point and it immediately moves on to the except statement. So the code following x = int(‘Choi’) is not executed.
try, except, else
The try, except, else statement is the addition of the else statement after except in the above try, except statement. If an error occurs within the try statement, the code within the except statement is executed, but if an error does not occur, it moves to the else statement and executes the code within it.
print("==== Program begins ====")
try:
x = int('29')
print(f'my age is {x}')
except:
print("==== Error, but program is still alive ====")
else:
print('==== No error occurred. ====')
print("==== Program Ends ====")
I took the code from the example above, changed it to x = int(’29’) to prevent an error, and added an else statement. Let’s take a look at what the results will be if you run the code.
==== Program begins ====
my age is 29
==== No error occurred. ====
==== Program Ends ====
Since no exception occurred, of course all the code in the try statement is executed, and then it moves on to the else statement and executes the code in it.
try, finally
The finally statement is a statement that is executed unconditionally regardless of whether an error occurs. If you use only the try finally statement without except, after an error occurs, only the finally statement will be executed and the program will terminate midway.
print("==== Program begins ====")
try:
x = int('Choi')
print(f'my name is {x}')
finally:
print("==== FINALLY ====")
print("==== Program Ends ====")
Looking at the results, you can see that the code in x = int(‘Choi’) is executed and an error occurs and the program should be terminated, but the code in the finally statement is executed and the program is terminated.
The most common use of the finally statement is to close files. If an open file must be closed unconditionally regardless of whether an error occurs or not, you must write code to close the file within the finally statement.
try, except, finally
If you’ve read this far, I believe you now know the roles of try, except, and finally. If so, let’s take a look at how the code runs if you use all three constructs.
print("==== Program begins ====")
try:
x = int('Choi')
print(f'my name is {x}')
except:
print("==== except ====")
finally:
print("==== FINALLY ====")
print("==== Program Ends ====")
==== Program begins ====
==== except ====
==== FINALLY ====
==== Program Ends ====
Looking at the results, an error occurs at x = int(‘Choi’), the code print(f’my name is {x}’) after that is omitted, and it moves on to the except statement, and regardless of whether an error occurs, It moves to the working finally statement and executes the code. After exiting all statements, the print(“==== Program Ends ====”) code is executed and the program ends.
try, except, else, finally
Finally, what happens if you use all the phrases you’ve learned so far? In this example, let’s look at two codes: one that generates an error and one that does not.
Code which error occurs
print("==== Program begins ====")
try:
x = int('Choi')
print(f'my name is {x}')
except:
print("==== except ====")
else:
print('==== No error occurred. ====')
finally:
print("==== FINALLY ====")
print("==== Program Ends ====")
==== Program begins ====
==== except ====
==== FINALLY ====
==== Program Ends ====
Code which error doesn’t occur
print("==== Program begins ====")
try:
x = int('29')
print(f'my age is {x}')
except:
print("==== except ====")
else:
print('==== No error occurred. ====')
finally:
print("==== FINALLY ====")
print("==== Program Ends ====")
==== Program begins ====
my age is 29
==== No error occurred. ====
==== FINALLY ====
==== Program Ends ====
Looking at the results above, basically the try and except statements are essential for exception handling. Optionally, if you want to execute other code separately in a situation where an exception does not occur, you can use the else statement. If there is code that must be executed after the try statement ends regardless of whether an exception occurs or not, you can write it in the finally statement.
Summary
- try – Write code that is likely to cause an error (required)
- except – Write code to be executed when an error occurs (required)
- else – Write code you want to execute separately when an error does not occur (optional)
- finally – Write code that should be executed after the code in the try statement ends regardless of whether an error occurs (optional)