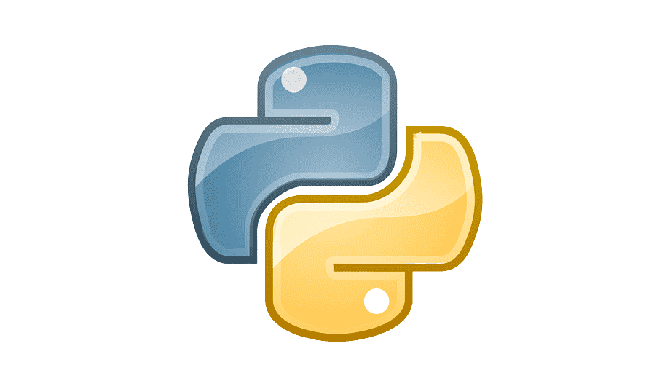
Python list slicing
Python list slicing refers to a method of extracting part of a list. Slicing is used to select multiple elements from a list and return the selected portions to a new list or use them for other purposes. Slicing is done by specifying a partial list using the index of the list. It can be used not only for lists but also for iterable objects (tuples, strings, etc.).
Basic syntax for list slicing
new_list = my_list[start:stop:step]
- start : slice start position (included)
- stop: Slice end position (not included, i.e. only from n – 1)
- step (optional): The step (interval) value. Specifies the interval to skip elements
Now that you know the basic syntax, let’s learn how to use it in detail by looking at various examples.
my_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing from 1 to 4 (select index 1 to 3)
sub_list = my_list[1:4] # sub_list = [1, 2, 3]
# Slicing from 2 to the end
sub_list = my_list[2:] # sub_list = [2, 3, 4, 5, 6, 7, 8, 9]
# Slicing with step 2 from first to 6 (select indices 0, 2, 4)
sub_list = my_list[:7:2] # sub_list = [0, 2, 4, 6]
# Slicing in reverse order
sub_list = my_list[::-1] # sub_list = [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
As mentioned when explaining the syntax, the stop part only includes up to n – 1, so in the case of my_list[1:4], only indexes 1, 2, and 3 are sliced. If you do not enter a number in start, it means starting from the beginning, and if you do not enter a number in stop, it means it starts until the end. Therefore, in the case of my_list[2:], slicing is performed from the 2nd index to the end. my_list[:7:2] means slicing by 2 spaces from the beginning to 6 (7 – 1). In the case of my_list[::-1], there are no numbers in the start and stop parts, so slicing is done from beginning to end. do. However, because the step (interval) is -1, slicing is performed from the end, ultimately returning an inverted list.
Slicing the entire list
my_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Copy a list using slicing (without changing the original list)
copy_of_list = my_list[:] # Create a copy of the original list in copy_of_list
If [:] start, stop, and step are not included as shown above, the entire list is sliced. In other words, it returns a list that is identical to the original list.
Slicing backwards from a specific section
my_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing in reverse order from index 4 to 1
sub_list = my_list[4:0:-1] # sub_list = [4, 3, 2, 1]
If the stop part is 0, index 0 is not included. Therefore, starting from the fourth index, 4, the step is -1, so slicing is done in reverse order up to index 1.