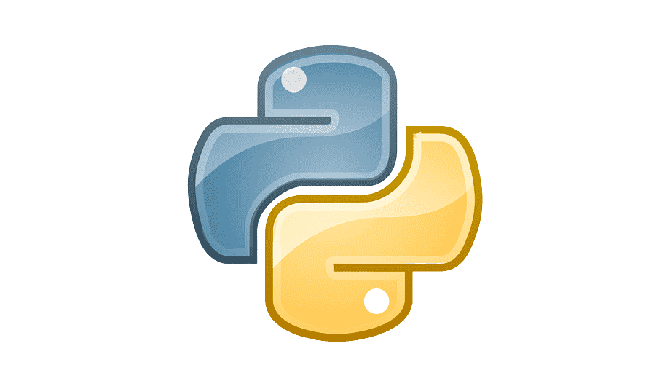
Python loops
In Python, you can execute a block of code multiple times using two types of loops. for and while. Today, let’s learn how to use each loop by looking at example codes.
for
The for loop iterates through the elements in a sequence (list, tuple, string, etc.) and immediately executes the code block.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
In the above code, the fruit variable points to each element in the list fruits one by one and executes the loop. The output result is as follows
apple
banana
cherry
You can also use range() function for counting numbers by using for loop. Let’s take a look an example code below.
# from 0 to 4
for i in range(5):
print(i)
When you learn programming, not just about Python, you should know that we count from 0, not 1, as we usually do, and the range() function doesn’t count the last number of a given number. Therefore, the code above performs counting 0 to 4 which is repeating 5 times.
Technically, In the range() function in the above code, it has given the number 5. Then it creates a sequence of numbers from 0 to 4 (5 is not included). This sequence is often used as an iterable in ‘for’ loops.
0
1
2
3
4
📢 Can i set start and end number?
That is actually a good question! You can set the start and end numbers by giving two numbers in order. Take an example code below.
# range(start, end)
for i in range(3, 10):
print(i)
In the above code, the first number is the start number, and the second number is the end number. Then the result will come out like this. Remember! The last number at the end is not included, so it will count until 9.
3
4
5
6
7
8
9
📢Okay, I get that the first number is the start and the second number is the end, but what if I want to set the size of each step?
Then you can give the third number in the range() function. The third number is for the size of each step. Let’s take a look at the example code below.
# start, end, step
for i in range(1, 10, 2):
print(i)
In this loop, range(1,10,2) is used to generate a sequence of numbers starting from 1, up to (but not including) 10, with a step size of 2,. So, the loop will iterate over the numbers 1,3,5,7 and 9.
1
3
5
7
9
Then, let’s take a look at the first example we have seen already, the fruit list example. We can also get the same result with the fruit list example by using the range() function.
fruits = ["apple", "banana", "cherry"]
# Using a for-each loop
for fruit in fruits:
print(fruit)
# Using a for loop with indexing
for i in range(len(fruits)):
print(fruits[i])
Both loops achieve the same result of printing each element of the fruits list, but they use different looping constructs to do so, The first loop is more concise and idiomatic in Python and is often preferred when you only need the elements of a list without their indices. The second loop gives you more control over the indices and is useful when you need both the elements and their indices.
apple
banana
cherry
apple
banana
cherry
while
In Python, a while loop is used to repeatedly execute a block of code as long as a specified condition is true. Here is the general syntax of a while loop.
while condition:
# Code block to be executed as long as the condition is true
The condition is a Boolean expression that is evaluated before each iteration of the loop. If the condition evaluates to True, the code block inside the loop is executed. After executing the code block, the condition is checked again. if it still evaluates to True, the loop continues to execute, if it evaluates to False, the loop terminates and the program continues executing the code after the loop.
📢Okay, I can see that ‘for’ and ‘while’ loops are for iterating, but I don’t see why there are two different types of loops. for what?
A for loop is typically used when you know beforehand how many times you need to iterate over a sequence or perform a certain task. It’s commonly used with iterable objects like lists, tuples, strings, etc.., where you want to perform some operation on each element of the sequence.
On the other hand, a while loop is used when you want to repeatedly execute a block of code as long as a specific condition is True, and you may not know in advance how many iterations will be needed. while loops are often used when the number of iterations depends on user input, the result of a computation, or some other dynamic condition.
Now, let’s take a look at simple example of using while loop that counts from 1 to 5
count = 1
while count <= 5:
print(count)
count += 1
The variable count is initialized to 1, and the loop continues as long as the count is less than or equal to 5. In the loop, count += 1 increments the value of count by 1 in each iteration. So the result would be like below.
1
2
3
4
5
It’s important to ensure that the condition inside the while loop will eventually become False, otherwise, you may end up with an infinite loop, which can cause your program to hang or crash. You can use control flows statements like break, continue or other mechanisms to manage the loop execution flow effectively.
Let’s take a look at example codes of using break and continue statements.
Using ‘break’ statement
count = 0
while True:
print(count)
count += 1
if count >= 5:
break
This loop will print numbers starting from 0 and incrementing by 1 in each iteration. However, when count reaches 5 or greater, the break statement is executed, causing the loop to terminate.
0
1
2
3
4
Using ‘continue’ statement
count = 0
while count < 5:
count += 1
if count == 3:
continue
print(count)
In this loop, when count equals to 3, the continue statement is executed, which skips the rest of the loop’s body for that iteration and moves on to the next iteration. So, the number 3 is not printed.
1
2
4
5
These examples demonstrate how break and continue can be used to control the flow of a while loop. ‘break‘ is used to exit the loop prematurely based on a condition, ‘continue‘ is used to skip the remaining code in the loop for the current iteration and proceed to the next iteration.