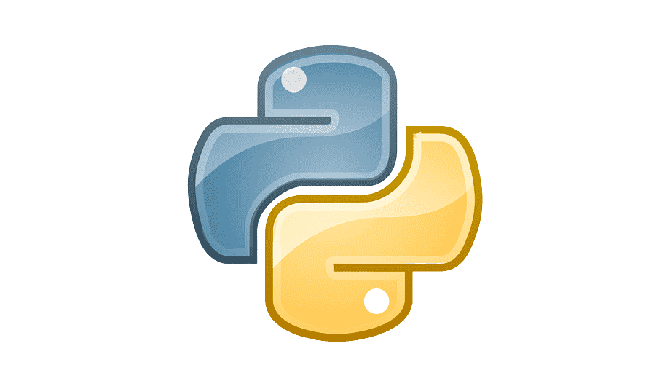
Python Variable
In Python, variables are named containers that store data during your program’s execution. They act like placeholders, allowing you to refer to specific values without constantly repeating them. Let’s take a look at some examples of variables and their ways of being used.
Creating and Naming
No need to declare them beforehand, just give them a name and assign a value like below example.
name = "Choi" # String variable
age = 30 # Integer variable
is_adult = True # Boolean variable
Technically, you can name whatever you want for variables, but there are some naming rules you have to follow. Check the naming rules below and remember when you name your variable.
- Start with a letter or underscore(_).
- Use letters, numbers or underscores after
- Be descriptive(ex : total_score instead of x)
- Case-sensitive : Name and name are DIFFERENT
Data Types
Variables can hold different types of data, and let’s see what types there are in variables.
# Numbers (integers, floats, complex numbers)
count = 10 # Integer
price = 9.99 # Float
complex_num = 3+2j # Complex number
# Strings (text)
message = "Hello, world!"
# Booleans (True or False)
is_valid = True
# Lists (ordered collections)
numbers = [1, 2, 3]
mixed_list = [10, "apple", True]
# Tuples (immutable lists)
coordinates = (3, 5)
# Dictionaries (unordered key-value pairs)
person = {"name": "Choi", "age": 30}
When you use variables, you have to consider their scope. There are only two types of variable scope, and let’s take a look below.
Variable Scope
- Local – Created inside functions or loops, only accessible within those blocks.
- Global – Defined outside functions, accessible everywhere(use cautiously).
def greet():
name = "Choi" # Local variable
print(f"Hello, {name}!")
greet() # "Hello, Choi!"
# name is not accessible here because it's local
age = 30 # age is accessible everywhere because it's global
Key Points
You can re-assign values to variables, changing their content
count = 5
count = count + 2 # Now count is 7
- Use operators like +, -, *, / to perform calculations on variables.
- Python is dynamically typed, no need to declare types beforehand.
- Variables are fundamental, master them for effective programming.